Show Success, Error, Warning messages in Magento 2
posted on
12/06/2018
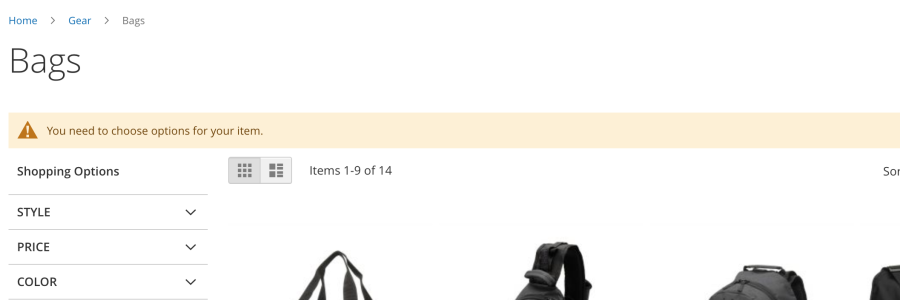
To be able to output warnings, error and notice messages in our modules we have to use the following interface \Magento\Framework\Message\ManagerInterface
.
The best way to use it is through Dependency Injection in the constructor as follows:
<?php
namespace VendorName\ModuleName\Block;
class MyClass
{
public function __construct(
\Magento\Framework\Message\ManagerInterface $messageManager
) {
$this->_messageManager = $messageManager;
}
Once this interface is added and initialized in the constructor, it becomes available to be used anywhere in the class, and we could use any of the following methods:
// Deprecated Methods
$this->messageManager->addSuccess()
$this->messageManager->addError()
$this->messageManager->addWarning()
$this->messageManager->addNotice()
Important: Magento has deprecated these methods in commit cd13a85f 18 May 2016
Instead, we should use the following methods:
// New Methods
$this->messageManager->addSuccessMessage()
$this->messageManager->addErrorMessage()
$this->messageManager->addWarningMessage()
$this->messageManager->addNoticeMessage()
Example:
$this->messageManager->addWrningMessage('You need to choose options for your item.')